Discover insights into the specifics of storefront notifications that Magento 2 uses to inform website visitors, as well as the existing best practices we recommend for Magento developers to follow and uses.
So, let’s get started by checking the interface. The message interface defines the following message types:
There is also an “exception” message which is tied to error messages and displayed on the storefront in the same color scheme.
Magento 2.1.0 release has deprecated the following methods of Manager Interface because they were not secure:
We recommend avoiding using these methods.
Instead of these methods, developers should use the following ones:
Additionally, new “complex” methods have been introduced:
Examples of usage
For better understanding in which cases a developer should use add<MessageType>Message, and when they should use similar complex methods let’s check the following examples.
Example #1
We need to display a “You deleted the address” text message when the address has been successfully removed from the customer’s address book.
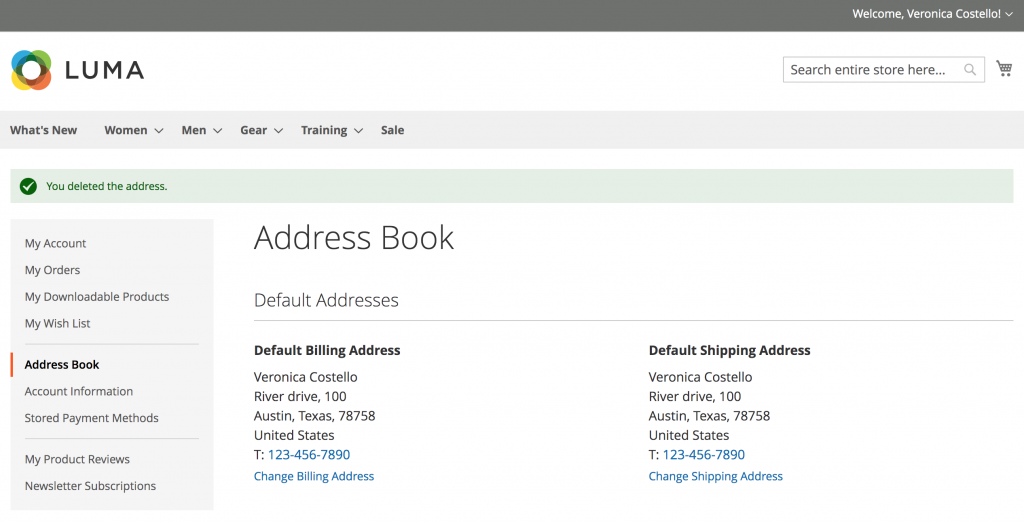
A text message that will be displayed on the storefront does not contain any special characters or HTML tags; therefore, we will use the addMessage method. And if we are talking about a successfully executed operation then in the add<MessageType>Message <MessageType> should be replaced with a Success word. As a result, we will get — addSuccessMessage:
$this->messageManager->addSuccessMessage(__('You deleted the address.'));
Note, that as an alternative option to display the mentioned message, a developer may use a more complicated approach:
$message = $this->messageManager ->createMessage(MessageInterface::TYPE_SUCCESS) ->setText( __('You deleted the address.') ); $this->messageManager->addMessage($message);
For example, we need to highlight an “address” word in the “You deleted the address” text message to be bold. In this case, a developer can use the next code:
$this->messageManager->addSuccessMessage(__('You deleted the address.'));
However, such approach will return the unexpected result and You deleted the <b>address</b>. message will be displayed on the storefront instead:
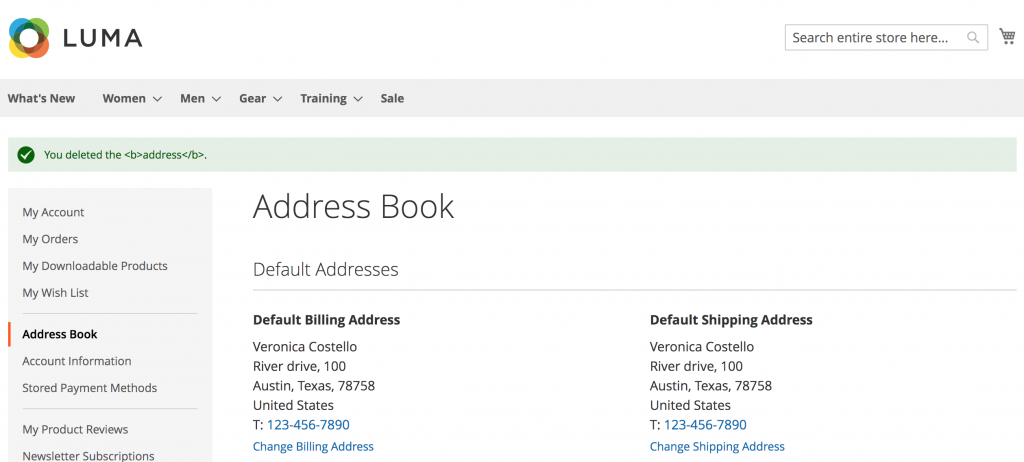
As you can see from the screenshot above addSuccessMessage is definitely not a way to display text messages containing HTML tags. For such cases developers must use a complex method, which is described in the next Example.
Example #2
In this example, we will examine a case where we need to display a text message that contains a link to the “customer/account/forgotpassword” pages. That’s a common case when a registered customer tries to register on the website again.
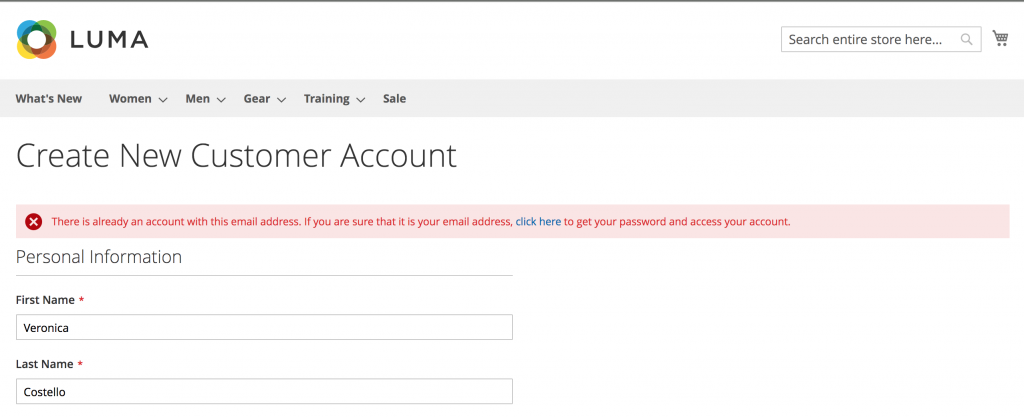
The next steps should be taken to display a message on the screenshot.
1. Create a template which will contain a necessary text message:
<?php /** * Copyright © Magento, Inc. All rights reserved. * See COPYING.txt for license details. */ /** @var \Magento\Framework\View\Element\Template $block */ ?> <?= $block->escapeHtml(__('There is already an account with this email address. If you are sure that it is your email address, <a href="%1">click here</a> to get your password and access your account.', $block->getData('url')), ['a']);
2. Use di.xml to add a new element into the message configuration pool:
... <type name="Magento\Framework\View\Element\Message\MessageConfigurationsPool"> <arguments> <argument name="configurationsMap" xsi:type="array"> <item name="customerAlreadyExistsErrorMessage" xsi:type="array"> <item name="renderer" xsi:type="const">\Magento\Framework\View\Element\Message\Renderer\BlockRenderer::CODE</item> <item name="data" xsi:type="array"> <item name="template" xsi:type="string">Magento_Customer::messages/customerAlreadyExistsErrorMessage.phtml</item> </item> </item> </argument> </arguments> </type> ...
3. Use message manager to call a customerAlreadyExistsErrorMessage message and set an URL to customer/account/forgotpassword page in Vendor/Module/Controller/Account/CreatePost.php:
... $this->messageManager->addComplexErrorMessage( 'customerAlreadyExistsErrorMessage', [ 'url' => $this->urlModel->getUrl('customer/account/forgotpassword'), ] ); ...
Note: check the code below to understand how a developer may call a customerAlreadyExistsErrorMessage message without addComplexErrorMessage method:
... $identifier = 'customerAlreadyExistsErrorMessage'; $message = $this->messageManager ->createMessage(MessageInterface::TYPE_ERROR, $identifier) ->setData( [ 'url' => $this->urlModel->getUrl('customer/account/forgotpassword'), ] ); $this->messageManager->addMessage($message); ...
Final inputs
- addExceptionMessage logs an exception error and can display an alternative error text to a user.
- There is no complex method for the addExceptionMessage unlike what we have with addComplexSuccessMessage for addSuccessMessage.
- If you want to use complex methods please avoid situations where you create a separate phtml template for a single text message.
Thank you for reading, and please don’t hesitate to post your questions if you have any.